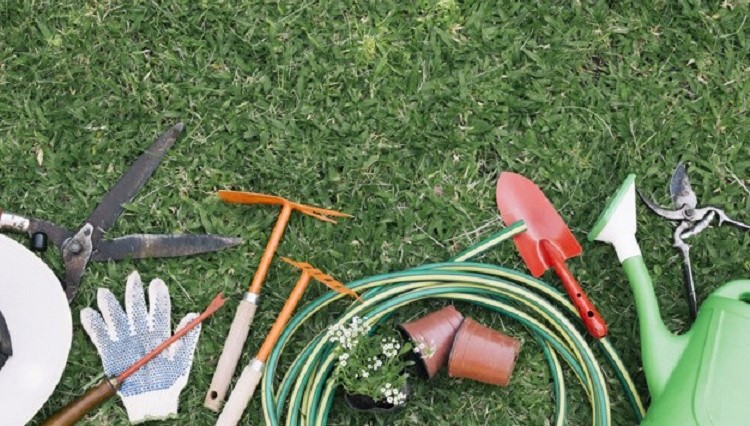
If you’ve been working with Angular, you might have found yourself in a situation where you questioned the need of using external data store libraries when it comes to state management architecture of your Angular application, especially if coming from the React world.
Recently, I stumbled upon one healthcare-related project having a few simple features like submitting an appointment, seeing appointments, etc. Basically, a CRUD application extended a bit. The project had NgRx incorporated… Guess what? I swear I had a feeling there was more NgRx boilerplate code than actual business code, and almost ended up switching my career to gardening… Obviously, that didn’t happen, this post has been born instead.
Disclaimer: The aim of this post is not to discard the usefulness of data store libraries, it’s about auditing whether built-in Angular tools can solve problems that external stores tend to solve, about their comparison. It’s also about discouragement of adopting stuff prior to realizing the actual utilization and need, and not following the hype blindly. It’s about not being a cargo cult programmer.
So, do I need external tools to manage the state of my Angular application?
To answer that question, it’s a good idea to rewind the clock and start from the origins of popularizing data store patterns in general, Facebook’s Flux (observer pattern slightly modified to fit React). Pete Hunt, one of the early contributors to React, says:
“You’ll know when you need Flux. If you aren’t sure if you need it, you don’t need it.”
Redux evolved on the Flux pattern. Kind of reduced version of the Flux with a single global store as the main difference. Dan Abramov, one of the creators of Redux says:
“I would like to amend this: don’t use Redux until you have problems with vanilla React.”
Visit When should I use Redux? for a reference.
Here is also Dan’s well-known article about the need for Redux: You Might Not Need Redux.
After a brief look at the history of data stores, we see that they originated in the React world to help cope with certain use-cases, but do the same problems from React.js apply to Angular as well?
One thing I love about Angular is that, unlike React.js (UI library), Angular is a complete framework. It offers everything you need from start to production-ready applications saving you from the hassle of looking into third-party libraries to solve specific architectural problems.
Ok, so does Angular have built-in mechanisms to solve the problems that Redux or similar stores were supposed to solve for React.js applications?
To answer that question we need to see what problems Flux, Redux, and stores in general solved or tend to solve:
“Bucket brigade” problem
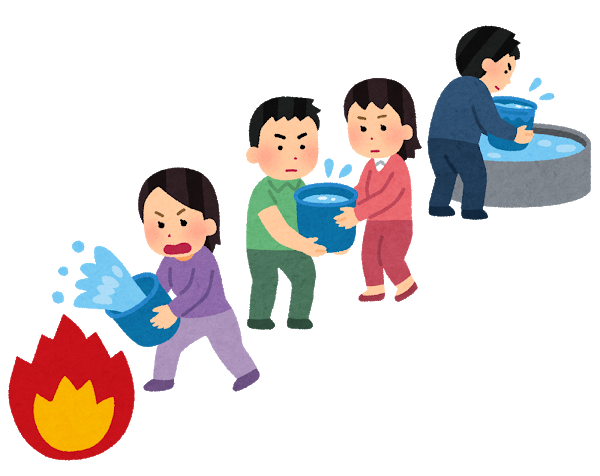
First, and one of the very main reasons Redux got widely used in the React world (although it wasn’t its primary function) is because it offered a way to cope with problems where the props would need to be passed deep down into the component tree, also called “prop drilling” problem. Those situations can occur from time-to-time, especially when dealing with complex applications. Communication between components in those situations can get really messy. Especially those components in the middle of the tree that act as a bridge only and don’t really consume those props can feel “extraneous”, at the same time becoming less reusable and more tied to the application.
That’s one side of the problem that Redux solved in React.js. Another one would be communication between interdependent components that are at completely different points in the component tree, i.e. non-related in terms of the component hierarchy.
The “prop drilling” issue applies to the Angular world as well, so is there an Angular way of coping with these problems?
As per the official Angular docs, one way of communication between components is using a shared service. Cool, so we don’t need an external library to solve the “prop drilling” issue in the Angular world?
Even better, thanks to the Hierarchical Dependency Injection in Angular, we can define the scope of the service visibility and make it visible only to the consuming hierarchy of the components. We don’t have to create a big global — application-level state for that purpose. It doesn’t really matter if that global state is immutable; it still exists and can create easy, subtle issues by not cleaning it up regularly.
Dependency Injection in Angular allows us to make local service, see dependency references, achieve modularity, decouple, and test things easier. Also, by associating service to certain parts of the components only, we don’t have to take care of cleaning the service and its eventual state as it will clean itself up once the components consuming the service get destroyed.
The shared service can always be easily provided at root hierarchical level if the need arises to share some state across the application.
As an aside, React.js also introduced Context API, a modern, simpler, lighter, and boilerplate-free solution to deal with the “prop drilling” issue.
States and Data Store
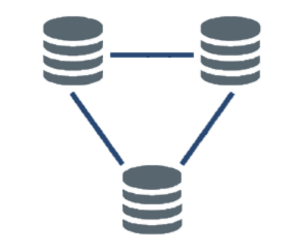
The application state is everything. Any action can be considered a state: opening dropdown, form filling, navigating to the page, triggering some work on the server, etc. Only a fraction of the applications can afford to persist all types of the states mainly using the components themselves, and from time to time, depending on the situation, you’ll need to manage the state in a more sophisticated way. That’s reasonable of course, and here we will go through some of the tools Angular offers to deal with managing state of an application:
A state machine called Angular Router. There are numerous and often overlooked advantages of using URL to manage the state of an application:
- Sharing/Storing the state of the application simply by sharing/storing the URL
- Not having to care about cleaning the state, state persists exactly as long as it should, yet with the ability to go back and forward at any point of the history using the browser navigation
- Functional browser history navigation
- Persisting the state on the application reload without having to deal with any type of storage (e.g. local storage)
Thanks to the child and auxiliary routes, and other powerful features Angular Router supports, URL seems like a perfect state manager candidate to consider for a great part of the applications. The caveat I can think of at the moment is the limitation of the URL length to 2000 characters in IE and Edge browsers (other major browsers support much more).
Note that Angular router is reactive and query parameters are Observable, meaning that whenever a query parameter changes all subscribers will be notified.
Still, in some cases managing the state using components and Router only isn’t sufficient, or it doesn’t fit the architecture of the application. Maybe you need to be able to preserve some specific state in the memory or local storage, cache some server responses. Whatever the reason is, again, we have powerful Angular built-in tools like services and RxJs.
Here is an example of observable data store service in Angular:
Note: Although we made our puppies immutable in this example, in Angular it’s essential only if we want to optimize the application performance to use OnPush
Change Detection strategy. It doesn’t necessarily bring other benefits (considering the trade-offs). More about that in the Immutability and Performance paragraph.
Simple, straightforward, boilerplate free (no actions, reducers, effects, selectors, dispatchers…), without scattering the related logic into multiple locations (it’s feature-based localized following the Angular’s official style guide). All by covering the fundamental benefits from common data store libraries such as the ability to notify all subscribers about the change, and principles such as a single source of truth, read-only state, immutability, etc.
We also made the store local/non-global, feature-based, visible only to the consuming hierarchy of the components, avoiding “stale data” (to make it global if needed, simply decorate it so it’s providedIn: 'root'
).
Using Angular DI, we can now also inject other depending stores, or inject and relate service responsible for communicating with the server.
As an aside, if the purpose of creating a client data store is caching server responses and syncing data on the client bypassing the server in order to spare some server resources, you may think again before committing to create one. Unless you have hundreds of thousands of users or some strict requirements, you should calculate trade-offs carefully, development is much more expensive than hardware. Syncing data on the client bypassing the server can be really ungrateful and cause subtle errors easily. Instead, try optimizing the server-client communication, let your server tell what data needs to be updated. This post has an interesting sight about, give it a read.
“There are only two hard things in Computer Science: cache invalidation and naming things” — Phil Karlton
Unidirectional Data Flow
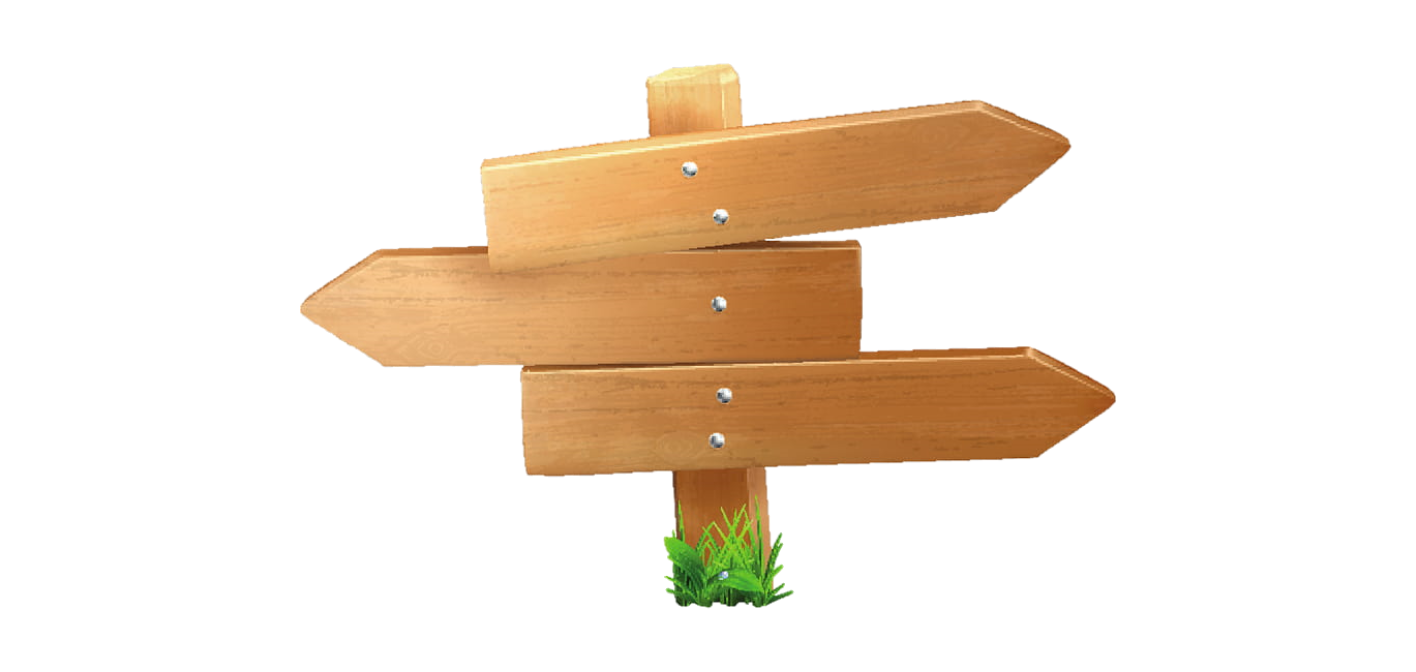
This was the main aim of Flux rather then Redux, and in the terms of Flux, it is described as preventing the view itself to dispatch further actions while rendering, and preventing other actions to be dispatched if the dispatch for an action is already ongoing.
This ensures the predictability of the properties and stability of the component tree. All by bringing great benefits to the performance.
Angular strictly enforces unidirectional data flow on the presentation layer (via input bindings), and prevents the view from updating itself out of the box. You must have come across the: “The expression has changed after it was checked” error if breaking the unidirectional data flow principle in Angular development mode.
Interestingly, Angular.js wasn’t enforcing unidirectional data flow and it’s one of the reasons it wasn’t quite performant. It was trying to stabilize the component tree using it’s famous “Digest Cycle” mechanism. For example, each time view updates itself a new cycle is run trying to stabilize the component tree with the limit of 10 iterations. Upon reaching the limit you’d see:
“10 $digest() iterations reached. Aborting!”.
Immutability and Performance
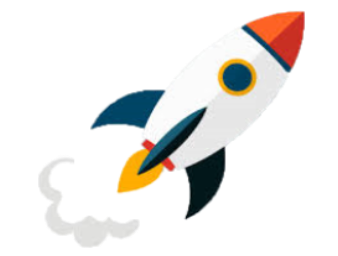
Depending on the need and use-case, keeping your data immutable may bring some benefits like predictability or easier mutation tracking, but when using some external data store it’s rather implied to not mutate the data that goes into the store. That’s reasonable of course; otherwise, one of the main benefits of a store like debugging would be lost. Additionally, the last thing you want is a single global mutable state.
Although immutability comes per se if you are using some external data store, you’d need to take care of keeping your data immutable yourself. That often seems to result in introducing additional tools to help you do that as it’s not always a trivial task, brings additional burden, and in some cases can cause GC and memory draining issues (more on this below).
But how does that relate to performance?
Performance is sometimes mentioned as a benefit of using external NgRx like data stores in Angular applications. But that’s only the case if you switch your application to use OnPush
Change Detection strategy. And the fact that immutability is enforced by data store patterns doesn’t simply mean you can turn your application to use OnPush
strategy out of the box — unless you keep absolutely all component inputs in the store, which seems like a huge overhead, especially if targeting the performance mainly.
“Premature optimization is the root of all evil.” — Donald Knuth
The Angular default Change Detection mechanism is blazing fast and optimized out of the box. Only the expressions bound in the templates are checked against changes — everything else is ignored. Considering that, in very few cases the application will actually benefit from OnPush
Change Detection strategy.
Still, if some specific part of the application is performance-wise critical, we can simply use OnPush
strategy without a store. Or in case of real-time charts, for example, it’s likely better to throttle the data, or even throttle the rendering by detaching/attaching the UI from Change Detectors tree.
Data stores and OnPush
Change Detection strategy can be used together but are not quite inherently linked.
Back to the immutability. Along with the additional effort to maintain your data immutable, a few cases have been reported here and there mentioning GC and memory draining issues caused by stores and immutability, narrowing the cases down to the large data sets or frequent store updates. In those cases, there is the recommendation to use Immutable.js or other libraries for copying the data more efficiently (e.g. by using structural sharing). But according to the comment on Dan’s answer here, it doesn’t seem to solve the problem either.
Having all of the above in mind, external data stores bringing performance benefits is questionable in the least and is rather strongly use-case related.
Debugging
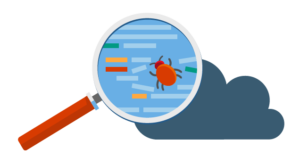
Debugging is often mentioned as one of the main benefits of having an external data stores like NgRx incorporated. While those debugging tools can be truly powerful, the need for actually using all those powers does not seem to arise that often.
RxJs has some handy debugging features available as well. Starting from the RxJs version 5, the debugging possibilities are extended, and what is currently available many will find quite sufficient. There are also plans to extend debugging possibilities further in future releases. For an essential how-to RxJs debugging visit this post.
Still, if you often find yourself in the need for hardcore debugging (should you question your codebase, then? 🤔), or the app is just that complex, there are also RxJs dev-tools available for debugging that offer a similar experience as with the tools that are shipped with some external data stores. Check rxjs-spy.
Conclusion
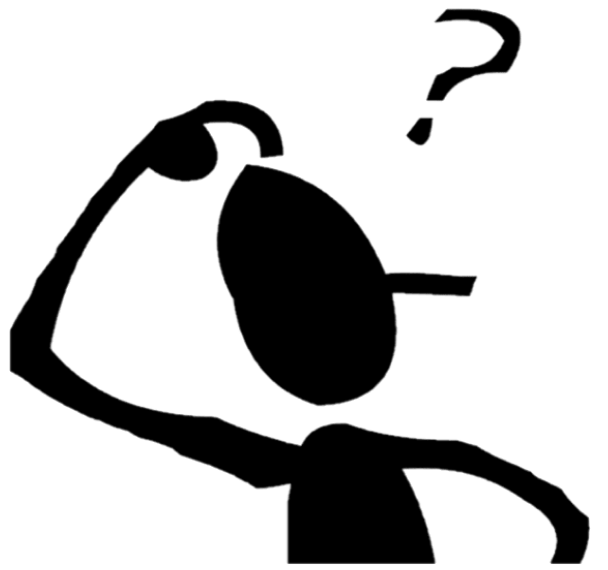
Data stores originated in React world to help cope with certain problems React.js as a UI library has faced at the time, and Angular community partly followed the trend recently, even though as a complete framework, Angular offers alternative, equal, or even superior built-in tools to deal with those certain problems.
Know your actual toolset before incorporating a new one. Don’t fix the problem you don’t have. Think about what best suits your architecture, requirements, and needs. Keep it simple. If a wild boar destroys your crops don’t buy a tank!
The trend with data stores seems to have gone a bit in the wrong direction in general. A lot of people seem to follow the hype and adopt it without even knowing the utilization, purpose, and actual trade-offs.
“I suppose it is tempting, if the only tool you have is a hammer, to treat everything as if it were a nail.” ― Abraham Maslow
Unless you’re building some highly interactive web game, or have hundreds of thousands of users and want to spare some server resources by keeping and syncing state on the client, you really should think twice before adopting some external client data store. There are always trade-offs involved. Depending on the use-case, the tool supposed to solve a problem can easily turn into a problem.
If you still feel the need of adopting some external data sore, take a look at NgRx alternatives gaining popularity like NgXs or Akita. Much less boilerplate code. NgXs feels more natural to the Angular ecosystem by incorporating the TypeScript decorators, and the learning curve is less steep.
Thank you for your interest.
All suggestions, corrections, and improvements are more than welcome and appreciated. ^^
Like!